Loose coupling or lose your mind
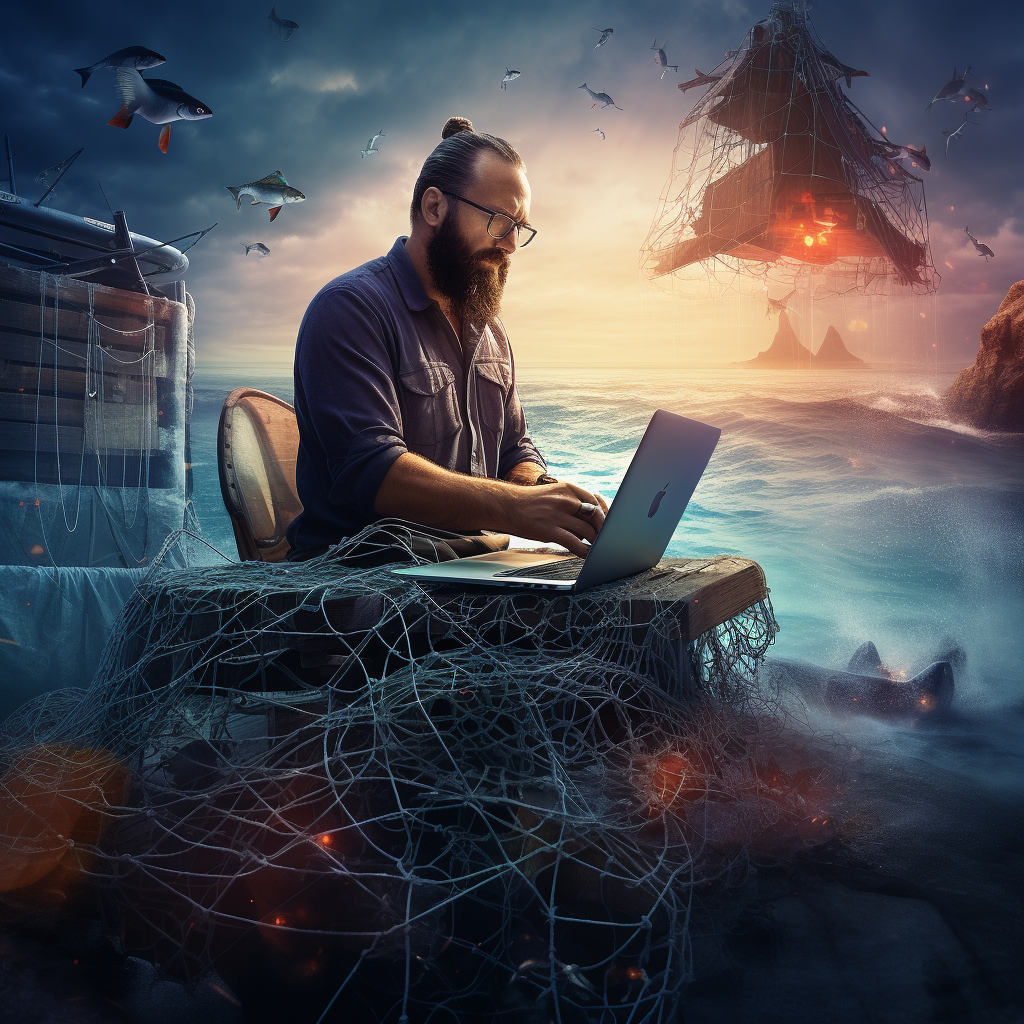
In the intricate world of software design, the principle of “loose coupling” serves as a guiding light, a method to ensure that the myriad components of a complex system can operate independently, like the graceful ballet of a well-rehearsed dance troupe. The absence of entangled dependencies allows for seamless interaction, where each component performs its role without causing a chain reaction of failures if one were to falter.
❓What is loose coupling?
Loose coupling is a measure of how tightly two or more software components are connected. In a loosely coupled system, components are connected through interfaces, which allow them to communicate and exchange data without being tightly integrated. This loose coupling makes the system more resilient to change and failure, as modifications to one component can be made without affecting others.
🪬 Why is loose coupling important?
There are several reasons why loose coupling is important in software design:
- Increased resilience: Loosely coupled systems are more resilient to failures, as a problem in one component doesn’t necessarily bring down the entire system.
- Enhanced modularity: Loosely coupled systems are easier to modularize, making them easier to develop, test, and maintain.
- Improved maintainability: Loosely coupled systems are easier to maintain, as changes to one component have a limited impact on others.
- Greater flexibility: Loosely coupled systems are more flexible, allowing them to adapt to changing requirements and technologies.
Decoupling can potentially reduce costs in the long term by increasing system modularity, which can lead to more manageable and efficient maintenance, better scalability, and easier integration of new features. By allowing parts of a system to be updated or fixed without impacting the whole, operational costs can be lowered. However, initial implementation of decoupling strategies might require significant investment in terms of development, infrastructure, and training. Therefore, while decoupling can offer cost savings, it should be approached with a clear understanding of both the upfront investment and the long-term benefits.
✨ Examples of loose coupling
Here are some examples of loose coupling in software design:
- A web application that uses a database: The web application and the database are loosely coupled, as they communicate with each other through an API.
- A microservices architecture: In a microservices architecture, each service is loosely coupled to other services, as they communicate with each other through APIs or messaging queues.
- A distributed system: In a distributed system, components are located on different machines and communicate with each other over a network.
- A library that uses a factory pattern: The library and the factory are loosely coupled, as the library does not know how the factory creates its objects.
- A component that uses an event bus: The component and the event bus are loosely coupled, as the component does not know how the event bus distributes events.
- A component that uses a configuration file: The component and the configuration file are loosely coupled, as the component does not know how the configuration file is structured.
🚀 Strategies for achieving loose coupling
There are many ways to achieve loose coupling in software design. Some common techniques include:
- Interface segregation principle states that clients should only depend on the interfaces that they actually use.
- Dependency inversion principle states that high-level modules should not depend on low-level modules but rather on abstractions.
- Message passing is a communication pattern in which components send messages to each other to exchange information. This allows components to communicate asynchronously and without knowing about each other’s implementation details.
- Event sourcing is a technique for storing events as a sequence of immutable records. This allows components to subscribe to events and react to them without knowing about each other’s internal details.
- CQRS stands for Command Query Responsibility Segregation. It is an architectural pattern that separates the concerns of reading data from the concerns of writing data. This can help to reduce coupling between components.
- Microservices are self-contained services that communicate with each other through APIs. This can help to break down large, monolithic applications into smaller, more manageable components.
- A layered architecture is an architectural pattern that divides an application into multiple layers. This can help to reduce coupling between layers and make the application more maintainable.
- Domain-driven design is an approach to software development that focuses on building a deep understanding of the domain problem. This can help to identify and decouple the concerns of different parts of the application.
📮 A collection of loose coupling patterns
Many different loose coupling patterns can be used in software design. Here is a small collection of some of the most common patterns:
- Dependency injection: This pattern allows components to receive their dependencies from a central location rather than creating them themselves.
- Composition over inheritance: This principle states that composing objects together is better than inheriting from them.
- Factory pattern: This pattern allows for creating objects without knowing their concrete implementation.
- Abstract factory pattern: This pattern extends the factory pattern to allow for the creation of families of related objects.
- Builder pattern: This pattern allows for the creation of complex objects step-by-step.
Loose coupling is a valuable principle in software design that can help to create more resilient, flexible, and maintainable systems. So, embrace loose coupling, my fellow coders. Let your code dance to the rhythm of independence, and watch your sanity soar like a majestic eagle. After all, a happy developer is a productive developer.